MVC e JQuery: datagrid con jqGrid
- Gregorat Paolo
- 15 lug 2016
- Tempo di lettura: 3 min
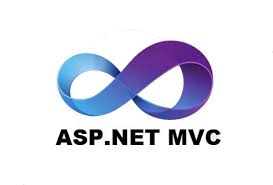
Oggi vi mostro un esempio di come poter inserire una grid jqGrid di JQuery in un'applicazione MVC
Nel nostro esempio recuperiamo i dati nel conroller tramite una chiamata ad una Web API che restituisce l'elenco delle Naziioni presenti nel suo DataBase
Al controller vengono passati i parametri codice e descrizione che ne permettono le ricerche filtrate per questi 2 campi presenti nella view sottoforma di textBox da poter compilare
Codice javascript da inserire nel file Nazioni.js
$(function () { $("#grid").jqGrid({ url: "/Nazioni/GetNazioni", datatype: 'json', mtype: 'POST', colNames: ['Id', 'Codice','Valuta','Descrizione'], colModel: [ { key: true, hidden: false, name: 'Id', index: 'Id', editable: true, width: 50 }, { key: false, hidden: false, name: 'Codice', index: 'Codice', editable: true, width: 120 }, { key: false, hidden: false, name: 'TBValuta.Codice', index: 'TBValuta.Codice', editable: true, width: 120}, { key: false, name: 'Descrizione', index: 'Descrizione', editable: true, width: 600 }], pager: jQuery('#pager'), rowNum: 10, rowList: [10, 20, 30, 40], height: '100%', viewrecords: true, caption: 'Lista Nazioni', postData: { Codice: function() { return $("#Codice").val(); }, Descrizione: function() { return $("#Descrizione").val(); } //Codice: $('#Codice').val(), //Descrizione: $('#Descrizione').val() }, emptyrecords: 'No records to display', jsonReader: { root: "rows", page: "page", total: "total", records: "records", repeatitems: false, Id: "0" }, autowidth: false, multiselect: false }).navGrid('#pager', { edit: true, add: true, del: true, search: false, refresh: true }, { // edit options zIndex: 100, url: '/Nazioni/Edit', closeOnEscape: true, closeAfterEdit: true, recreateForm: true, afterComplete: function (response) { if (response.responseText) { alert(response.responseText); } } }, { // add options zIndex: 100, url: "/Nazioni/Create", closeOnEscape: true, closeAfterAdd: true, afterComplete: function (response) { if (response.responseText) { alert(response.responseText); } } }, { // delete options zIndex: 100, url: "/Nazioni/Delete", closeOnEscape: true, closeAfterDelete: true, recreateForm: true, msg: "Are you sure you want to delete this task?", afterComplete: function (response) { if (response.responseText) { alert(response.responseText); } } }); });
function ReloadGrid() { $("#grid").jqGrid("setGridParam", { datatype: "json" }) .trigger("reloadGrid", [{ current: true }]); }
Codice da inserire nella view Nazioni.cshtml
@using DemoClientWebMVC.Models @model NazioniSearchViewModel
@{ ViewBag.Title = "Nazioni"; }
<h2>Gestione Nazioni</h2>
<div class="row">
<div class="col-md-8"> <section id="nazioniForm" class="active"> @using (Html.BeginForm("Nazioni", "Nazioni", new { ReturnUrl = ViewBag.ReturnUrl }, FormMethod.Post, new { @class = "form-horizontal", role = "form" })) { <div> <h3>Filtri</h3> </div>
<div class="form-group"> @Html.LabelFor(m => m.Codice, new { @class = "col-md-2 control-label" }) <div class="col-md-10"> @Html.TextBoxFor(m => m.Codice, new { @class = "form-control" }) @*@Html.TextBox("codice", null, new { @class = "form-control" })*@ </div> </div>
<div class="form-group"> @Html.LabelFor(m => m.Descrizione, new { @class = "col-md-2 control-label" }) <div class="col-md-10"> @Html.TextBoxFor(m => m.Descrizione, new { @class = "form-control" }) @*@Html.TextBox("descrizione", null, new { @class = "form-control" })*@ </div> </div>
<div class="form-group"> <div class="col-md-offset-2 col-md-10"> <input id="btnReload" onclick="ReloadGrid()" type="button" value="Cerca" class="btn btn-default"/> </div> </div>
} </section> </div> </div>
<div> <hr width="100%" /> </div>
<div> <div class="form-group"> <table id="grid" width="400"></table> <div id="pager"></div> </div> </div>
<link href="~/Content/themes/base/jquery.ui.all.css" rel="stylesheet" type="text/css" /> <link href="~/Content/jquery.jqGrid/ui.jqgrid.css" rel="stylesheet" type="text/css" /> <script src="~/Scripts/jquery-1.10.2.min.js" type="text/javascript"></script> <script src="~/Scripts/jquery-ui-1.10.4.min.js"></script> <script src="~/Scripts/i18n/grid.locale-en.js" type="text/javascript"></script> <script src="~/Scripts/jquery.jqGrid.min.js" type="text/javascript"></script> <script src="~/Scripts/Nazioni.js" type="text/javascript"></script>
Codice da inserire nel controller Nazioni.cs
using Newtonsoft.Json; using Newtonsoft.Json.Linq; using NovaDTO; using System; using System.Collections.Generic; using System.Linq; using System.Net; using System.Net.Http; using System.Web.Http; using System.Web.Mvc;
namespace DemoClientWebMVC.Controllers { public class NazioniController : Controller { public ActionResult Index() { return View(); }
public JsonResult GetNazioni( string Codice, string Descrizione, string sidx, string sord, int page, int rows) //Gets the todo Lists. { int pageIndex = Convert.ToInt32(page) - 1; int pageSize = rows; if (string.IsNullOrEmpty(MyGlobalVariables.PathServer)) return null;
string requestString = MyGlobalVariables.PathServer + "/odata/Nazione?$expand=TBValuta($select=Codice)&$select=Id,Codice,Descrizione";
int numFiltri = 0; if (!string.IsNullOrEmpty(Descrizione)) { requestString += "&$filter=startswith(tolower(Descrizione), '" + Descrizione.ToLower() + "')"; numFiltri++; }
if (!string.IsNullOrEmpty(Codice)) { if (numFiltri == 0) //requestString += "&$filter=tolower(Codice) eq '" + txt_Codice.Text.ToLower() + "'"; requestString += "&$filter=startswith(tolower(Codice), '" + Codice.ToLower() + "')"; else requestString += " and startswith(tolower(Codice), '" + Codice.ToLower() + "')";
numFiltri++; }
string token = MyGlobalVariables.Token;
HttpResponseMessage response = Utility.WebAPI.RunGetCallWithToken(requestString, token); if (response.IsSuccessStatusCode) { string elencoStr = response.Content.ReadAsStringAsync().Result; var jsonResult = JsonConvert.DeserializeObject<JObject>(elencoStr); var todoListsResults = JsonConvert.DeserializeObject<List<TBNazioneDTO>>(jsonResult.GetValue("value").ToString(), new JsonSerializerSettings { ReferenceLoopHandling = ReferenceLoopHandling.Ignore }).AsEnumerable(); //return Json(res, JsonRequestBehavior.AllowGet);
int totalRecords = todoListsResults.Count(); var totalPages = (int)Math.Ceiling((float)totalRecords / (float)rows); if (sord.ToUpper() == "DESC") { todoListsResults = todoListsResults.OrderByDescending(s => s.Descrizione); todoListsResults = todoListsResults.Skip(pageIndex * pageSize).Take(pageSize); } else { todoListsResults = todoListsResults.OrderBy(s => s.Descrizione); todoListsResults = todoListsResults.Skip(pageIndex * pageSize).Take(pageSize); } var jsonData = new { total = totalPages, page, records = totalRecords, rows = todoListsResults }; return Json(jsonData, JsonRequestBehavior.AllowGet); } else return null;
}
[System.Web.Mvc.HttpPost] public string Create([Bind(Exclude = "Id")] TBNazioneDTO objTodo) { return ""; } public string Edit(TBNazioneDTO objTodo) { return ""; } public string Delete(int Id) { return "Deleted successfully"; }
}
}
Codice da inserire nel file Site.css
.dropdown-submenu{position:relative;} .dropdown-submenu>.dropdown-menu{top:0;left:100%;margin-top:-6px;margin-left:-1px;-webkit-border-radius:0 6px 6px 6px;-moz-border-radius:0 6px 6px 6px;border-radius:0 6px 6px 6px;} /*.dropdown-submenu:hover>.dropdown-menu{display:block;}*/ .dropdown-submenu>a:after{display:block;content:" ";float:right;width:0;height:0;border-color:transparent;border-style:solid;border-width:5px 0 5px 5px;border-left-color:#cccccc;margin-top:5px;margin-right:-10px;} .dropdown-submenu:hover>a:after{border-left-color:#ffffff;} .dropdown-submenu.pull-left{float:none;}.dropdown-submenu.pull-left>.dropdown-menu{left:-100%;margin-left:10px;-webkit-border-radius:6px 0 6px 6px;-moz-border-radius:6px 0 6px 6px;border-radius:6px 0 6px 6px;}
Comments